While Loop That Reads in Yes or No to Continue
Continue in C++ While loop
Continue statement:
Inside the loop, the continue statement is used to control the loop.
C++ utilizes the continue keyword to implement the continue statement, which transfers the program's flow at the start of the loop and skips the current statement when it is reached. Continue statement is used in all the iteration control loops.
SYNTAX:
The syntax of the continue statement is: continue;
- Continue is a predefined keyword.
- The continue statement can't be used outside of the iteration loop. It only appears in iteration statements.
Flowchart:
The following image shows the flow chart of continue statements in loops:
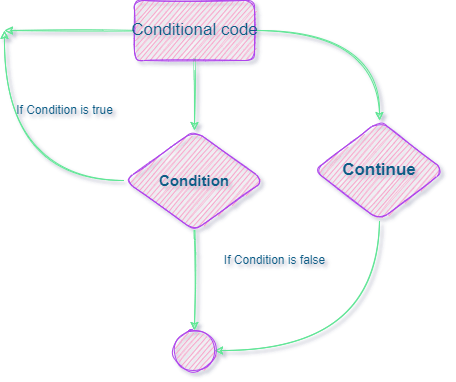
Explanation:
The statements within the loop are executed based on the loop condition. If the specified condition is true, the loop iteration will be continued to execute and the loop is terminated when the specified condition is false. The continue statement skips the current statement and passes control to the next loop iteration when the continue keyword is used inside a loop.
While Loop
A while loop or while statement repeats all code of its body as long as a specific condition is satisfied. The loop ends if or when the condition no longer met.
The syntax for the while loop:
The syntax for a while loop is given by:
while(condition) { statement(x); }
Usage of Continue Statement in While Loop:
When the continue is used in the While loop the control immediately jumps to the Boolean expression.
while () { { // ... } continue; }
Example 1: Program to print the list of numbers
Algorithm:
Step 1: Start the program
Step 2: Read n, i
Step 3: Initialise i=1
Step 4: iterate until I<=10 if the condition is false then go to step 4
Step 5: Print the value of I,
If (i==3)
Continue;
Else
go to step 4
Step 6: Stop.
#include<iostream> using namespace std; int main() { int i, n; cout << "Enter a number"; cin >> n; cout << "the numbers are"; i=1; while(i <= n) { if(i == 3) { continue; } cout << i; i++; } return 0; }
The output of the program:
Enter a number: 8 The numbers are: 1 2 4 5 6 7 8
Explanation:
The program is designed to print the list of numbers which are entered by the user.
We have used the While loop method in the above code to demonstrate the implementation of the continue statement.
We've set up two variables: "n" for entering the value and "i" for the iteration. The program asks the user for a number, which is then stored in n. We initialize i to 0(i=0) in the while loop and add a condition that verifies that "i" is less than or equal to n (i<=n).
We used the continue statement inside the loop to skip number three. If the number is not 3, the statement outside the 'if condition' is executed, and the number is printed one by one.
Example 2: Program to print the following numbers:
#include <iostream> using namespace std; int main() { int j=10; while (j >=0) { if (j==6) { j--; continue; } cout<<" the Value of j: "<<j<<endl; j--; } return 0; }
The output of the program:
The value of j: 10 The value of j: 9 The value of j: 8 The value of j: 7 The value of j: 5 The value of j: 4 The value of j: 3 The value of j: 2 The value of j: 1
Explanation:
The While loop method was used in the above program to demonstrate the implementation of the continue statement.
The program is designed to print a list of numbers. We have used a variable "j" for the iteration. We initialized j to 10 (j=10) in the while loop and specified a condition that verifies that "j" is more than or equal to n ( j >= n). We used the continue statement inside the loop to skip the number six. If the number is not 6, the statement outside the if condition is executed, and the number is printed one by one.
Continue with the Nested loop:
When the continue keyword is used with nested loops, the current iteration of the inner loop is skipped.
Program:
// using continue statement inside // nested while loop #include <iostream> using namespace std; int main() { int number, i, j; int sum = 0; // nested while loops // first loop i=1; while(i<=3) { // second loop j=1; while(j<=3) { j++; if(j==2) { continue; } cout << "i = " << i << ", j = " << j << endl; } i++; } return 0; }
Output of the program:
i = 1, j = 2 i = 1, j = 4 i = 1, j = 5 i = 2, j = 2 i = 2, j = 4 i = 2, j = 5 i = 3, j = 2 i = 3, j = 4 i = 3, j = 5 i = 4, j = 2 i = 4, j = 4 i = 4, j = 5
Explanation:
When the continue statement is used in the above program, it skips the current iteration of the inner loop. And the program's control shifts to the update expression of inner loop. As a result, the value of j=3 never appears in the output.
ferrellovelinterst.blogspot.com
Source: https://www.tutorialandexample.com/continue-in-cpp-while-loop
0 Response to "While Loop That Reads in Yes or No to Continue"
Post a Comment